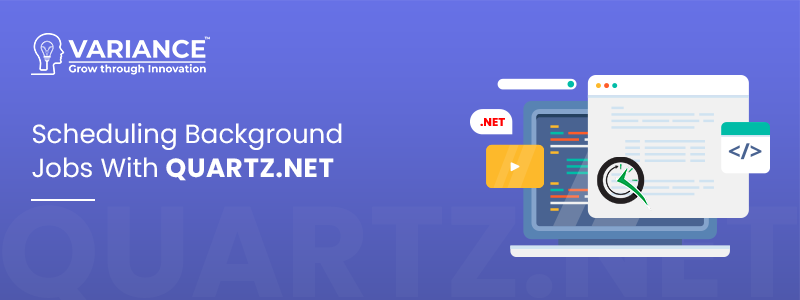
Topics covered in this article are…
- What is Quartz.Net?
- Installing Quartz.Net
- How to Add Quartz.Net hosted service?
- How to create a IJob?
- Configuration of the Job with a cron schedule
- Configure the Job in appsttings.json
- Configure the Job in appsttings.json
- Conclusion
What is Quartz.Net?
Quartz.NET is a library for .NET developers that helps them schedule and automate tasks within their applications. It’s like having a digital scheduler that can perform specific jobs at specific times or on a recurring basis.
For example, think of it as an alarm clock app for your computer. You can set it to remind you to take a break every hour, send an email every morning, or run a backup process every night.
With Quartz.NET, developers can easily set up and manage tasks like these in their applications, saving time and effort by automating repetitive jobs. It’s a handy tool for improving efficiency and ensuring important tasks get done on time.
In Quartz.NET, the main concepts revolve around scheduling and executing jobs. Here are the key concepts in Quartz.NET:
- Job: A job represents a task or unit of work that needs to be executed. It is defined by implementing the IJob interface, which requires the implementation of the Execute method. Jobs are the building blocks of the tasks you want to schedule and automate.
- Trigger: A trigger defines the schedule for when a job should be executed. It determines the timing and frequency of job execution. There are various types of triggers available, such as SimpleTrigger (for one-time execution), CronTrigger (for more complex schedules using Cron expressions), and more.
- Scheduler: The scheduler is the central component of Quartz.NET that manages the execution of jobs based on their triggers. It is responsible for starting, stopping, and rescheduling jobs as per their defined schedules.
Quartz.NET is a tool that helps you schedule tasks, like running jobs, at specific times in your application. To handle the scheduling part, you can use the Quartz.NET hosted service. This service takes care of running tasks in the background of your application. It constantly checks for triggers that are set to fire and run the associated jobs as needed.
To use Quartz.NET, you first need to set up the scheduler. But after that, you don’t have to worry about starting or stopping it. The IHostedService takes care of managing the scheduler for you.
Our project structure look like this:
Installing Quartz.Net:
To get started with Quartz .NET, you need to install the necessary components. Follow these steps:
- Open your project in your development environment, like Visual Studio.
- Go to the “Package Manager Console” or “Manage NuGet Packages” menu.
- In the search bar, type “Quartz” and look for the “Quartz” and “Quartz.Extensions.Hosting” package.
- Click on the “Install” button to add the Quartz .NET package to your project.
That’s it! Now you have Quartz .NET installed in your project, and you can start using it to schedule and manage tasks in your application.
When you open the .csproj file of your project, you’ll see something similar to the following content:
How to Add Quartz.Net hosted service:
The Quartz.NET hosted service in an .NET application is straightforward. The hosted service takes care of running scheduled jobs in the background. Here’s a simple and beginner-friendly explanation along with the necessary code:
- Open your .NET project in Visual Studio or your preferred development environment.
- In the DemoTask/Program.cs file, locate the ConfigureServices method. This is where you configure services for your application.
- To register the required services with the Dependency Injection (DI) container. This step allows Quartz.NET to work seamlessly with other parts of your application, making it easy to manage and schedule jobs. By registering these services, you enable Quartz.NET to utilize the DI container to create and manage jobs effectively.
- Inside the ConfigureServices method, add the following code to register the Quartz.NET hosted service:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureServices((hostContext, services) =>
{
// Register the Quartz.NET hosted service
services.AddQuartz(options =>
{
// Use a Scoped container to create jobs (this allows using scoped services in your jobs)
options.UseMicrosoftDependencyInjectionScopedJobFactory();
});
// Set this to true if you want Quartz.NET to wait for jobs to complete during shutdown
services.AddQuartzHostedService(options => options.WaitForJobsToComplete = true);
// Other services and configuration can be added here
});
}
- UseMicrosoftDependencyInjectionScopedJobFactory: The UseMicrosoftDependencyInjectionScopedJobFactory is a feature in Quartz.NET that allows you to create jobs using the Dependency Injection (DI) container with a scoped lifetime. Using the UseMicrosoftDependencyInjectionScopedJobFactory, you can take advantage of the DI container’s scoping capabilities and ensure that each job gets its own scoped instance of services, preventing any conflicts or resource leaks.
- WaitForJobsToComplete: The WaitForJobsToComplete is a feature in Quartz.NET that determines how the application handles running jobs when it is shutting down or stopping. WaitForJobsToComplete to true is generally a safer approach, as it allows your application to handle jobs’ completion correctly before shutting down. It ensures that all scheduled tasks are completed, maintaining the application’s data integrity and preventing any unexpected behavior during shutdown.
Now, when you run your application, you’ll notice that the Quartz service starts running, and it will show a bunch of messages on the console:
How to create an IJob:
Creating an IJob in Quartz.NET is quite simple. An IJob is an interface that defines the task or job that you want to execute. To create an IJob, follow these steps:
Step 1: Create a new class that implements the IJob interface.
Step 2: In the Execute method of your MyDemoJob class, write the logic for the job you want to run. The IJobExecutionContext parameter contains information about the job execution context, but in this simple example, we’re not using it.
Step 3: That’s it! Your DemoTask/Job/MyDemoJob.cs class now represents the job you want to execute, and it’s ready to be scheduled using Quartz.NET.
using Quartz;
public class MyDemoJob : IJob
{
public Task Execute(IJobExecutionContext context)
{
// Your job logic goes here
// For example, you can perform some tasks or operations
// that you want to run on a scheduled basis.
Console.Out.WriteLineAsync("Executing my background job");
return Task.CompletedTask;
}
}
Now you can use this IJob implementation to schedule and run your job at specified intervals or triggers in your application.
Configuration the Job with a cron schedule:
To configure the job and schedule it in the Program.cs file using Quartz.NET, follow these steps:
- First, make sure you have created your MyDemoJob class that implements the IJob interface (as shown in the previous response).
- Open the DemoTask/Program.cs file in your .NET application.
- Inside the ConfigureServices method, add the necessary code to configure and schedule the job.
using DemoTask.Job;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Quartz;
using Quartz.Impl;
using Quartz.Spi;
using System;
using static Quartz.Logging.OperationName;
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureServices((hostContext, services) =>
{
// Add the required Quartz.NET services
services.AddQuartz(option =>
{
// Use a Scoped container to create jobs
option.UseMicrosoftDependencyInjectionScopedJobFactory();
// Configure and schedule the job
option.AddJob<MyDemoJob>(j => j.WithIdentity("MyDemoJob").Build());
// Trigger the job to run every 5 seconds
option.AddTrigger(t => t
.WithIdentity("MyDemoJobTrigger")
.ForJob("MyDemoJob")
.WithCronSchedule("0/5 * * * * ?")
);
});
// Add the Quartz.NET hosted service with WaitForJobsToComplete set to true
services.AddQuartzHostedService(option => option.WaitForJobsToComplete = true);
// Other services and configuration can be added here
});
}
- AddJob: AddJob is a method provided by Quartz.NET that allows you to register and add a job to the Quartz scheduler. When you use AddJob, you are defining the task that you want to execute as part of your job.
- AddTrigger: AddTrigger is a method provided by Quartz.NET that allows you to create and configure a trigger for your job. A trigger specifies when and how often the job should be executed. You can set the trigger’s schedule using various options like a specific time, a repeated interval, or a Cron expression to define complex schedules.
- WithCronSchedule: In simple terms, WithCronSchedule is a method provided by Quartz.NET that allows you to create a trigger with a schedule defined using a Cron expression. A Cron expression is a powerful way to specify complex and flexible time-based schedules for running your job. WithCronSchedule, you can set up your job to run at specific times, on certain days of the week, or at regular intervals that match your unique requirements. This level of granularity and flexibility makes it easy to control when your job should be executed, allowing you to design intricate job scheduling patterns.
In the above code, we use services.AddQuartz to configure the Quartz.NET services. We register the MyDemoJob using AddJob and set an identity for it. Then, we add a trigger for the job using AddTrigger, specifying its identity and a simple schedule that triggers it to run every 5 seconds.
Lastly, we add the Quartz.NET hosted service with WaitForJobsToComplete set to true, ensuring that jobs are completed gracefully during application shutdown.
Once you run your application, you’ll notice the familiar startup messages appearing as usual. After that, you’ll see something new every 5 seconds. The `MyDemoJob` will write “Executing my background job” to the console repeatedly.
Configure the Job with appsettings.json:
make your Quartz.NET job scheduling more flexible, you can extract the job schedule to the appsettings.json file. This allows you to change the schedule easily without modifying your application’s code. Here’s how you can do it:
- Open your DemoTask/appsettings.json file and add the schedule configuration under the “Quartz” section. For example:
{
"Quartz": {
"JobSchedule": "0/5 * * * * ?"
}
}
In this example, the JobSchedule property is set to “0/5 * * * * ?”, which represents the Cron expression for running the job every 5 seconds.
- In the ConfigureServices method in your DemoTask/Program.cs file, retrieve the schedule from the configuration:
using DemoTask.Job;
using Microsoft.Extensions.Configuration;
using Quartz;
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureServices((hostContext, services) =>
{
IConfiguration configuration = hostContext.Configuration;
// Add the required Quartz.NET services
services.AddQuartz(option =>
{
option.UseMicrosoftDependencyInjectionScopedJobFactory();
// Register the MyDemoJob with a schedule from appsettings.json
string jobSchedule =
configuration.GetValue<string>("Quartz:JobSchedule");
option.AddJob<MyDemoJob>(j => j.WithIdentity("MyDemoJob").Build());
option.AddTrigger(t => t
.WithIdentity("MyDemoJobTrigger")
.ForJob("MyDemoJob")
.StartNow()
.WithCronSchedule(jobSchedule)
);
});
services.AddQuartzHostedService(option => option.WaitForJobsToComplete = true);
// Other services and configuration can be added here
});
}
When you run the application again, you’ll notice that it produces the same output as before. The job will keep writing to the output every 5 seconds, just as we configured it.
Conclusion:
In conclusion, Quartz.NET offers a convenient and efficient solution for scheduling jobs in .NET applications. By using Quartz.NET, you can easily create and manage background tasks, set custom schedules, and control job execution with triggers. The support for Dependency Injection simplifies job creation, allowing you to use scoped services seamlessly.
With Quartz.NET, you can schedule jobs to run at specified intervals or times, making your application more dynamic and responsive. The flexibility of using Cron expressions for scheduling provides fine-grained control over job execution.
Overall, Quartz.NET is a powerful tool that enhances the functionality of your application by automating tasks, improving productivity, and delivering a reliable job scheduling solution. Whether it’s simple recurring tasks or complex workflows, Quartz.NET is a valuable addition to your .NET projects.
Do You Need more information?
For any further information / query regarding Technology, please email us at info@varianceinfotech.com
OR call us on +1 630 861 8283, Alternately you can request for information by filling up Contact Us







