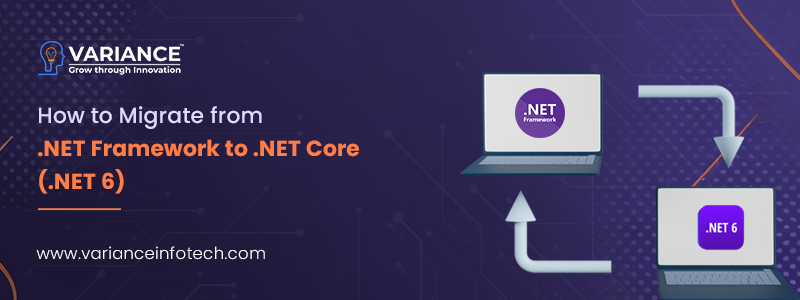
Introduction:
The evolution of .NET continues with the arrival of .NET 8, marking a pivotal moment for developers invested in the Microsoft ecosystem. This transition from the traditional .NET Framework to the cutting-edge .NET 8 represents not just a version upgrade but an essential leap toward embracing modernization, performance enhancements, and new features. In this blog, we’ll explore the intricacies of migrating your applications from .NET Framework to .NET 8, providing actionable guidance for a successful transition using below index:
- Transition Tools
- Manual Transitioning
- Dependency Analysis
- Preparing for transitioning
- Project File transition
- Code Rectification & Building
- Application Testing on .NET 6
- Unavailable Technologies in .NET Core
- Utilizing the .NET Portability Analyzer
- Recap
1. Transition Tools:
Microsoft provides a tool called the “.NET Upgrade Assistant” that helps make the migration process from .NET Framework to .NET Core simpler. This tool automates many tasks involved in the migration, making the overall process easier.
You can download the migration tool, “.NET Upgrade Assistant,” by following the instructions provided here:
Additionally, there’s another tool called “try-convert” available on GitHub. It’s a straightforward tool designed to assist in migrating projects based on .NET Framework to .NET Core.
Both tools aim to streamline the migration process by automating various tasks, making it more manageable for users.
2. Manual Transitioning:
For intricate projects that might encounter issues during migration with automated tools or for those seeking more precise control over the migration process, opting for manual migration is an alternative. This method involves a step-by-step manual migration process devoid of automation. The manual migration typically occurs across five key steps outlined below:
2.1 Dependency Analysis:
Before commencing your migration process, it’s crucial to conduct a comprehensive analysis of all third-party dependencies utilized by your applications. This analysis ensures that these dependencies are compatible with .NET 6 or have suitable alternatives available, such as newer versions of the same package or other packages offering similar functionalities. It’s important to note that exploring alternatives may involve additional efforts in terms of coding and testing, requiring a deliberate decision-making process.
For each referenced package, it’s essential to verify its compatibility with .NET 6. You can check this compatibility via the NuGet Gallery and examine the package dependencies. Packages with dependencies on .NET Standard are expected to be compatible with .NET 5+ since .NET supports all versions of the .NET Standard.
In .NET, it’s feasible to rely on the .NET Framework package. However, if you opt for this approach, thorough and extensive testing of your .NET 6 application becomes imperative to preempt any unexpected issues.
Once you’ve ensured that all your third-party dependencies align well with .NET 6, you can proceed to the next step in the migration process.
2.2 Preparing for Transitioning:
Transitioning the packages.config file: In .NET Framework applications, references to external packages are stored in the packages.config file. However, this file isn’t compatible with .NET Core-based projects. In .NET 6, project files use the PackageReference property to specify NuGet packages for applications. Therefore, during the transition, the references from the packages.config file in .NET Framework need to be transferred to the project file in .NET 6. This can be done manually or through the “Migrate packages.config to PackageReference” option available in Visual Studio.
API Compatibility during Transitioning: APIs available in both .NET Framework and .NET Core differ. It’s essential to thoroughly check the APIs used in the .NET Framework-based application against those available in .NET 6. Modify APIs that aren’t supported in the target framework (.NET 6). Additionally, consider targeting your .NET Framework-based application to at least .NET Framework 4.7.2 to ensure access to the latest API alternatives, especially where .NET Standard doesn’t support the APIs used in the .NET Framework-based project. To access a significant portion of the .NET Framework API in .NET Core, consider adding a reference to the Microsoft.Windows.Compatibility NuGet package.
Transitioning from App.Config to appsettings.json: .NET Framework projects typically use the App.config file for configuration, which needs to be replaced with appsettings.json in .NET Core projects.
Utilizing the .NET Portability Analyzer tool: This tool is beneficial for identifying APIs used in your project that aren’t present in .NET Core, aiding in the transitioning process.
By following these steps and making the necessary adjustments, you can effectively prepare your .NET Framework project for transitioning to .NET 6, ensuring a smoother shift and compatibility with the new environment.
2.3 Project File Transition:
In transitioning to .NET 6, it’s crucial to note the differences in project file formats between .NET 6 and .NET Framework-based projects. The newer .NET 6 projects utilize the SDK-style project file format. You have the choice to create a new project file in the required format or modify the existing project file to adhere to the SDK-style format.
For maintaining designer support within your project, you can opt to create a new .NET project in parallel with the old one and share assets between them. This allows modifications to UI elements in the old project through the corresponding Visual Studio environment. As assets are shared, any changes made in the old .NET Framework-based project will reflect in the new .NET 6 project as well.
AssemblyInfo Considerations: In the newer .NET 6 project, Attributes are autogenerated. If the .NET Framework-based project contains an AssemblyInfo.cs file, it might lead to duplicated definitions, causing compiler conflicts. To mitigate this issue, either disable autogeneration of Attributes or delete the older AssemblyInfo.cs file.
Disabling autogeneration of Attributes can be achieved in the .NET project file by adding the following entries:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
</PropertyGroup>
</Project>
Resource Migration: While embedded resources are included automatically, regular resources are not. Therefore, migrating resources from the .NET Framework-based project to the new project file is necessary.
Updating Package References: Lastly, update the version numbers of packages required in the new .NET project, ensuring compatibility as identified in the earlier “Prepare for Migration” step.
By following these steps, adjusting project file formats, managing resources, and updating package references, you’ll facilitate a smoother transition from the .NET Framework to .NET 6, minimizing compatibility issues and ensuring the project’s readiness for the new environment.
2.4 Code Rectification & Building:
Transitioning a .NET Framework application to .NET 6 involves a meticulous process of code rectification, updating dependencies, and adapting the application to align with the latest framework standards. This transformation ensures compatibility, optimization, and the utilization of new features provided by .NET 6.
During this transition journey, developers encounter challenges in refactoring legacy code, handling namespace changes, incorporating Dependency Injection (DI), and updating MVC components. These steps are crucial for a seamless transition and ensuring the application’s functionality remains intact post-transition.
Namespace Changes:
When transitioning from .NET Framework to .NET Core, several namespace alterations become pivotal. These changes reflect the restructured architecture and enhancements within the .NET Core framework. Below are examples showcasing common namespace transitions you’d encounter during this shift:
System.Web namespace removal:
// .NET Framework
using System.Web.Mvc;
using System.Web.UI;
// Other System.Web.* namespaces
// .NET Core
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Http;
// Other Microsoft.AspNetCore.* namespaces
Here,
System.Web.Mvc
and other System.Web.*
namespaces are replaced with Microsoft.AspNetCore.Mvc
, Microsoft.AspNetCore.Http
, and other related Microsoft.AspNetCore.*
namespaces in .NET Core for ASP.NET functionalities.Logging Namespace Transition:
// .NET Framework
using System.Diagnostics;
// .NET Core
using Microsoft.Extensions.Logging;
The replacement of
System.Diagnostics
in .NET Framework with Microsoft.Extensions.Logging
in .NET Core focuses on logging functionalities.Entity Framework Namespace Transition:
// .NET Framework
using System.Xml;
// .NET Core
using System.Xml;
// No significant changes observed in this namespace
The shift from
System.Data.Entity
in .NET Framework to Microsoft.EntityFrameworkCore
in .NET Core caters to Entity Framework functionalities.Configuration Namespace Changes:
// .NET Framework
using System.Configuration;
// .NET Core
using Microsoft.Extensions.Configuration;
In this case,
System.Configuration
in .NET Framework has been replaced with Microsoft.Extensions.Configuration
in .NET Core for handling configurations.Logging Namespace Changes:
// .NET Framework
using System.Data.Entity;
// .NET Core
using Microsoft.EntityFrameworkCore;
Here,
System.Data.Entity
in .NET Framework has been replaced with Microsoft.EntityFrameworkCore
in .NET Core for Entity Framework functionalities.
The list above outlines some common namespace transitions, but the specific dependencies and functionalities of your project might necessitate additional adjustments in namespaces.
Dependency Injection (DI):
Need toIdentify legacy code sections that directly instantiate services or have hardcoded dependencies. Refactor these sections to implement Dependency Injection, facilitating better testability, maintainability, and extensibility. Consider following example:
Legacy Code (Without DI):
public class LegacyService
{
// Legacy service without DI
public string GetData()
{
// Legacy logic
return "Legacy data";
}
}
public class LegacyController : Controller
{
private readonly LegacyService _legacyService;
public LegacyController()
{
_legacyService = new LegacyService(); // Hardcoded instantiation
}
public IActionResult Index()
{
var data = _legacyService.GetData();
return View(data);
}
}
Refactored Code (With DI):
public interface INewService
{
string GetUpdatedData();
}
public class NewService : INewService
{
public string GetUpdatedData()
{
// Updated logic
return "Updated data";
}
}
public class NewController : Controller
{
private readonly INewService _newService;
public NewController(INewService newService)
{
_newService = newService; // Injected via constructor
}
public IActionResult Index()
{
var data = _newService.GetUpdatedData();
return View(data);
}
}
This demonstrates a basic conversion from a legacy approach to utilizing Dependency Injection in a .NET 6 application.
Remember, the transition process may vary depending on the complexity of the application and the specific features and dependencies used. Testing and validation are crucial at each step to ensure functionality is maintained after the transition.
Configuration Changes:
To transit configuration settings from web.config to appsettings.json or environment variables. Use the
IConfiguration
interface to access configuration settings in .NET 6. Refer following example:// Accessing configuration in a class
public class MyClass
{
private readonly IConfiguration _configuration;
public MyClass(IConfiguration configuration)
{
_configuration = configuration;
}
public void MyMethod()
{
var settingValue = _configuration["MySettingKey"];
// Use the setting value
}
}
Middleware and Startup Changes:
ASP.NET Core Middleware manages HTTP requests and responses. Here’s how you can update Middleware configurations. For this consider the example of logging middleware:
In .NET Framework, logging might have been managed differently, possibly through libraries like
System.Diagnostics
or log4net.
In .NET 6 with ASP.NET Core, the built-in logging middleware is available by default, and you can configure it in the
Startup.cs
file.Example of setting up logging middleware in
Startup.cs
:public class Startup
{
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ILogger<Startup> logger)
{
// Other configurations...
app.UseRouting();
// Add logging middleware
app.Use(async (context, next) =>
{
logger.LogInformation("Handling request: " + context.Request.Path);
await next.Invoke();
logger.LogInformation("Finished handling request.");
});
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
// Other endpoints...
});
}
}
This example demonstrates a custom middleware that logs information for each incoming request and outgoing response.
Startup Configuration Changes
The
Startup.cs
file in ASP.NET Core is the entry point that configures services and the application’s request handling pipeline.Example: Configuring Services
In .NET Framework, service configuration might have been handled in the
Global.asax.cs
file or via other custom initialization methods.In .NET 6 with ASP.NET Core, service configurations are typically done in the
For any further information / query regarding Technology, please email us at info@varianceinfotech.in
OR call us on +1 630 534 0220, Alternately you can request for information by filling up Contact Us
ConfigureServices
method within the Startup.cs
file.
Example of configuring services in
Startup.cs
:public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddScoped<IMyService, MyService>();
// Other service registrations...
}
}
Here, services like Controllers and custom services (IMyService) are added to the dependency injection container.
Explanation: The Startup.cs file in ASP.NET Core is pivotal for configuring the application’s request handling pipeline, encompassing services, middleware, and endpoints. This transition involves moving configurations from Global.asax or similar methods to Startup.cs counterparts. Middleware configuration involves integrating custom logic or built-in components into the request processing pipeline, with the order of middleware addition being critical for handling requests and responses effectively. For instance, provided examples demonstrate middleware that logs information during request-response cycles, while service configuration registers dependencies within the application’s dependency injection container. These changes signify a shift from the traditional .NET Framework approach towards application startup, routing, and middleware handling to the more modular and adaptable approach in ASP.NET Core, particularly notable in .NET 6.
This breakdown covers essential steps for migrating both API and MVC web applications to .NET 6, addressing specific changes like Dependency Injection and the removal of the System.Web namespace. Each step requires careful consideration and thorough testing to ensure a successful migration.
2.5 Application Testing on .NET 6:
Absolutely! Once you’ve meticulously gone through all the preceding steps and made the necessary adjustments in your project for the transition from .NET Framework to .NET 6, it’s essential to conduct rigorous testing to verify that the code performs as anticipated. This crucial testing phase validates the success of your .NET Framework to .NET Core porting process.
Running and testing your code thoroughly serves as the litmus test to ensure:
- Functionality: Check that all the features and functionalities of your application operate as expected in the .NET 6 environment.
- Compatibility: Verify that the code interacts seamlessly with the new .NET 6 framework without encountering compatibility issues.
- Performance: Assess the performance metrics to confirm that the application behaves optimally and efficiently in the new environment.
- Edge Cases: Test various scenarios, including edge cases, to catch any potential errors or unexpected behavior.
By conducting comprehensive testing, you’ll ascertain the successful transition of your application from .NET Framework to .NET 6, ensuring its stability, functionality, and compatibility in the new environment. This step significantly contributes to a smooth and successful migration, providing confidence in the updated application’s reliability and performance.
3. Unavailable Technologies in .NET Core:
Here’s a breakdown of technologies present in .NET Framework that aren’t available in .NET Core or .NET 5+:
- AppDomains: The capability to create application domains is not supported in .NET Core.
- Remoting: Communication across application domains using remoting is no longer supported in .NET Core.
- Code Access Security (CAS): CAS, a sandboxing technique replaced by Security Transparency in .NET Framework 4.0, is not supported in .NET Core.
- Windows Communication Foundation (WCF) Server: WCF, a framework for building service-oriented applications, is not supported in .NET Core (.NET 5+).
- Windows Workflow Foundation (WF): WF, a framework for building workflow-enabled applications, is not supported in .NET Core (.NET 5+).
- System.EnterpriseServices: COM+ functionality is also not supported in .NET Core (.NET 5+).
These technologies from the .NET Framework are not directly available or supported in .NET Core or later versions. Developers transitioning their applications should consider alternative approaches or frameworks available in the .NET Core or .NET 5+ ecosystem to achieve similar functionalities or re-architect their solutions to adapt to the supported technologies in the new environment.
4. Utilizing the .NET Portability Analyzer:
Here’s a step-by-step demonstration of using the .NET Portability Analyzer tool to check the compatibility of a .NET Framework-based project:
Prerequisites:
- Download and install the Visual Studio extension for .NET Portability Analyzer. It’s available for Visual Studio 2017, 2019 and 2022.
Steps:
- Open Visual Studio 2019 and navigate to Extensions -> Manage Extensions.
- Search for “.NET Portability Analyzer” and click Download.
- Close Visual Studio to complete the installation (ensure no instance of Visual Studio is running during installation).
Using the .NET Portability Analyzer:
- Open Visual Studio 2019 and navigate to Extensions -> Manage Extensions.
- Search for “.NET Portability Analyzer” and click Download.
- Close Visual Studio to complete the installation (ensure no instance of Visual Studio is running during installation).
Using the .NET Portability Analyzer:
- After installation, restart Visual Studio 2019.
- Open the project “ProCodeGuide.Samples.PDFSharp” downloaded from the GitHub Repository.
- Right-click on the project file in the Solution Explorer.
- You should see two new options: “Portability Analyzer Settings” and “Analyze Project Portability”.
- Select Portability Analyzer Settings to choose the target .NET Core platforms/versions for evaluation.
- After selecting the target platform, right-click on the project again and choose Analyze Project Portability.
- This action runs the analysis on the selected project, generating a report.
- The generated report has three sections:
- Portability Summary: Shows the portability percentage for each assembly. It may display 100% for the project assembly in .NET 5.0.
- Details: Provides a detailed report for each type used in the assembly, indicating whether they’re supported in the target framework (.NET 5.0 in this case).
- Unresolved Assembly: Lists assemblies referenced or used by the project but not analyzed by the .NET Portability Analyzer. Resolve dependencies according to the Manual Migration process mentioned earlier
By following these steps, you can install and utilize the .NET Portability Analyzer tool to assess the compatibility of your .NET Framework-based project with the target framework (.NET Core/.NET 6). This analysis helps identify potential issues and ensures a smoother migration process.
5. Recap:
Absolutely, opting for the manual approach during the transition from .NET Framework to .NET Core (.NET 6) offers distinct advantages. With the manual option:
- Control Over Code: You have better control and visibility into the modifications made to the application code. This allows for precise adjustments and ensures a deeper understanding of the changes being implemented.
- Project File Customization: Transitioning project files manually allows for tailored adjustments to align with the new SDK-style project format used in .NET 6. This flexibility ensures that project files are optimized for the new environment.
- Granular Dependency Management: By handling dependencies manually, you can perform a more detailed examination of third-party dependencies. It facilitates the identification of compatible alternatives or modifications necessary for a smooth transition.
- Fine-tuning Compatibility: By manually modifying the codebase, you can specifically address API differences between .NET Framework and .NET Core (.NET 6), ensuring seamless compatibility.
- Enhanced Understanding: Through hands-on adjustments, developers gain a deeper understanding of the transition process and the intricacies involved, contributing to better learning and skill development.
Overall, the manual approach empowers developers with greater control, deeper insight, and a more comprehensive understanding of the changes necessary for a successful transition. While it may involve more effort initially, the benefits in terms of customization and understanding often outweigh the automated transition’s convenience.
Do You Need more information?
For any further information / query regarding Technology, please email us at info@varianceinfotech.in
OR call us on +1 630 534 0220, Alternately you can request for information by filling up Contact Us
Leave a Reply