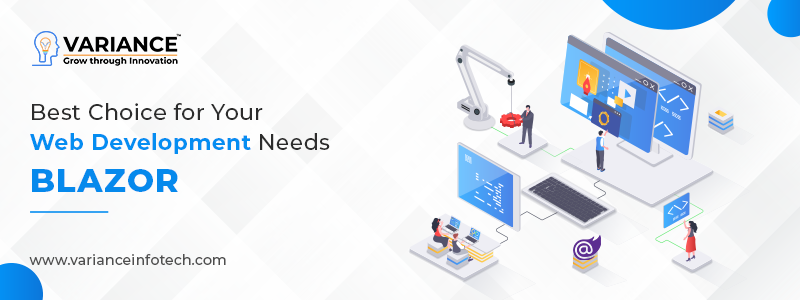
Introduction:
In the realm of web development, the emergence of Blazor represents a transformative leap, redefining conventions with its innovative approach. Developed by Microsoft, Blazor introduces a groundbreaking shift by enabling developers to construct dynamic web applications using the familiar language of C# and the robust framework of .NET. Unlike traditional web frameworks heavily reliant on JavaScript, Blazor offers a paradigm where the entire web application can be crafted using C#, bridging the gap between frontend and backend development seamlessly. This transformative capability arises from Blazor’s two hosting models: Blazor Server and Blazor WebAssembly. The former orchestrates application logic on the server side, facilitating real-time updates, while the latter delivers the entire application to the client’s browser using WebAssembly, ensuring high performance akin to native applications.
The adoption of Blazor heralds a new era in web development,, where developers wield the power of a unified ecosystem. This is exemplified by the integration of frontend and backend logic within a single language, eliminating the need for language-switching complexities. Moreover, Blazor’s reliance on a component-based architecture empowers developers to create reusable UI components, fostering scalability, maintainability, and code reusability. Its compatibility with the extensive .NET ecosystem further amplifies its appeal, offering seamless integration with existing libraries, tools, and packages. Additionally, the performance and security enhancements brought forth by Blazor WebAssembly, with its near-native execution speed and C#’s inherent static typing, ensure not only high performance but also fortified application security.
Benefits of Using Blazor:
Single Language for Frontend and Backend:
This benefit of Blazor is a significant advantage, allowing developers to utilize C# for both client-side and server-side development. This unification streamlines the development process, reduces the learning curve for different languages, and enhances code reusability. Here’s an in-depth look at this benefit with code examples:
Blazor Server:
Shared Language
Blazor Server employs C# as the primary language for developing both frontend and backend functionalities. Developers proficient in C# can seamlessly transition their skills to building interactive web applications without the need to learn additional languages like JavaScript for frontend work.
Code Reusability
In Blazor Server, methods written in C# on the server-side can be seamlessly utilized in the frontend Blazor components. Consider the following example:
Shared C# method in a service class:
// Shared C# method in a service class
public class SharedService
{
public string GetGreeting()
{
return "Hello, World!";
}
}
This
GetGreeting()
method can be consumed both on the server-side and the client-side within a Blazor component:@inject SharedService sharedService
<h1>@sharedService.GetGreeting()</h1>
Here, the
GetGreeting()
method defined in a C# class (SharedService
) is injected into a Blazor component, enabling code reuse between the backend and frontend in a Blazor Server application.Managing Data Consistency
In Blazor Server applications, data models defined in C# on the server-side can be directly utilized in the frontend Blazor components. This facilitates maintaining data consistency throughout the application.
For example, consider a data model defined in C# on the server-side:
// C# data model
public class User
{
public int Id { get; set; }
public string Name { get; set; }
// Other properties...
}
This same
User
model can be employed in a Blazor component for UI interactions:@code {
private User currentUser = new User { Id = 1, Name = "John Doe" };
}
<h2>Welcome, @currentUser.Name!</h2>
Here, the
User
class defined in C# on the server-side is utilized directly within a Blazor component to manage and display user information, ensuring data consistency across the application.Blazor Web Assembly:
Shared Language
Similar to Blazor Server, Blazor WebAssembly also uses C# for both frontend and backend development, bringing uniformity to the entire application stack.
Code Reusability
In Blazor WebAssembly, C# code can be shared between the server and the client by defining shared code libraries or using shared projects. For example:
Shared code library in a Blazor WebAssembly project:
public static class SharedUtility
{
public static string GetMessage()
{
return "Hello, World! From WebAssembly";
}
}
Utilizing the shared code in a Blazor WebAssembly component:
@code {
private string message = SharedUtility.GetMessage();
}
<h1>@message</h1>
Here, the
SharedUtility
class with the GetMessage()
method is used within a Blazor WebAssembly component to display a message, showcasing code sharing between the server and client in a Blazor WebAssembly application.Managing Data Consistency
Similarly, in Blazor WebAssembly, shared data models written in C# can be used both on the server-side and the client-side. This maintains data consistency throughout the application stack.
For instance, a shared data model in a Blazor WebAssembly project:
// Shared C# data model
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
// Other properties...
Using the shared data model in a Blazor WebAssembly component:
@code {
private Product currentProduct = new Product { Id = 1, Name = "Blazor Awesome" };
}
<h2>Current Product: @currentProduct.Name</h2>
In this example, the
Product
class defined in C# is utilized within a Blazor WebAssembly component to manage and display product information, ensuring consistency in the representation of data.Full Stack .NET Development:
This aspect in Blazor refers to its ability to leverage the entire .NET ecosystem, enabling developers to utilize .NET for both frontend (client-side) and backend (server-side) aspects of web development. This allows for a seamless integration of various .Net functionalities, tools, and libraries across the entire application stack. Let’s explore this feature in detail with code examples and explanations:
Blazor Server:
Unified Ecosystem
In a Blazor Server application, you can seamlessly use .NET libraries and tools on both the server-side and client-side. For instance, using a simple example of utilizing a .NET library within a Blazor component:
@using System.Net.Http // Importing a .NET namespace
@code {
private string serverResponse;
private async Task FetchData()
{
using var client = new HttpClient();
serverResponse = await client.GetStringAsync("https://api.example.com/data");
}
}
<button @onclick="FetchData">Fetch Data</button>
<p>@serverResponse</p>
Here, the
HttpClient
class from the System.Net.Http
namespace, which is part of the .NET framework, is used within a Blazor Server component to make an HTTP request and display the response.Database Integration
Blazor Server applications can utilize Entity Framework Core for database interactions. Here’s an example of fetching data from a database using Entity Framework Core within a service:
// Example of a Blazor Server service using Entity Framework Core
public class DataService
{
private readonly ApplicationDbContext _context;
public DataService(ApplicationDbContext context)
{
_context = context;
}
public async Task<List<Item>> GetItems()
{
return await _context.Items.ToListAsync();
}
}
This service can then be injected into a Blazor Server component to fetch and display data from the database.
Blazor Web Assembly:
Unified .NET Ecosystem
In Blazor WebAssembly, you can also leverage .NET libraries on the client-side. For example, working with JSON serialization using a .NET library:
@code {
private string serializedData;
private void SerializeObject()
{
var data = new { Name = "John", Age = 30 };
serializedData = System.Text.Json.JsonSerializer.Serialize(data);
}
}
<button @onclick="SerializeObject">Serialize Data</button>
<p>@serializedData</p>
This example showcases the usage of
System.Text.Json.JsonSerializer
from the .NET framework for JSON serialization within a Blazor WebAssembly component.Database Integration
While direct database access from Blazor WebAssembly is limited due to security reasons, interactions with databases are typically performed through backend APIs or services. Here’s an example of calling a backend API built with .NET technologies:
@inject HttpClient httpClient
@code {
private string serverResponse;
private async Task FetchData()
{
serverResponse = await httpClient.GetStringAsync("https://api.example.com/data");
}
}
<button @onclick="FetchData">Fetch Data</button>
<p>@serverResponse</p>
Here, the
HttpClient
is used to make an HTTP request to a backend API endpoint built using .NET technologies, retrieving data to be displayed within the Blazor WebAssembly component.Component-Based Architecture:
This feature in Blazor is a fundamental concept that allows developers to create reusable and encapsulated UI components, enhancing the maintainability, reusability, and scalability of web applications. Let’s explore this aspect in Blazor Server and Blazor WebAssembly with code examples:
Blazor Server:
Component-Based Structure
In Blazor Server, components are the building blocks of the UI. They encapsulate both UI markup and the logic associated with it. For instance, creating a simple reusable component:
<!-- Example of a Blazor Server component -->
<h3>GreetingsComponent</h3>
<p>@Message</p>
@code {
[Parameter]
public string Message { get; set; }
}
This
GreetingsComponent
can be used in other parts of the application by passing a message to it:<GreetingsComponent Message="Hello, World!" />
This demonstrates how components encapsulate UI elements and can be reused across different parts of a Blazor Server application.
Component Composition
Blazor Server allows for the composition of larger components from smaller, reusable components. For instance, creating a more complex component by nesting the
GreetingsComponent
within another:<!-- Example of a composed Blazor Server component -->
<h2>WelcomeComponent</h2>
<div>
<GreetingsComponent Message="Hello, Blazor!" />
</div>
Here, the WelcomeComponent
includes the GreetingsComponent
within it, showcasing how smaller components can be composed to create more complex and reusable structures.
Blazor Web Assembly:
Component-Based Structure
Similarly, in Blazor WebAssembly, components play a pivotal role in creating modular and reusable UI elements. For instance, creating a reusable button component:
<!-- Example of a Blazor WebAssembly component -->
<button @onclick="OnClick">@ButtonText</button>
@code {
[Parameter]
public string ButtonText { get; set; }
[Parameter]
public EventCallback OnClick { get; set; }
}
This
ButtonComponent
can be utilized across various parts of a Blazor WebAssembly application:<ButtonComponent ButtonText="Click me!" OnClick="@HandleClick" />
@code {
private async Task HandleClick()
{
// Handle click logic
}
}
Component Composition
Blazor WebAssembly also supports component composition, allowing developers to create more complex UI structures by combining smaller components. For example:
<!-- Example of composed Blazor WebAssembly component -->
<LayoutComponent>
<HeaderComponent />
<MainContentComponent />
<FooterComponent />
</LayoutComponent>
Here, the
LayoutComponent
composes smaller components like HeaderComponent, MainContentComponent
, and FooterComponent
to form a complete layout structure.High Performance:
This benefit in Blazor refers to its ability to deliver responsive and efficient web applications, leveraging optimizations to ensure rapid rendering and execution. This advantage is evident in both Blazor Server and Blazor Web Assembly. Let’s explore the High-Performance benefits in both contexts:
Blazor Server:
Efficient Rendering
Using SignalR, Blazor Server provides real-time communication between the server and the client, enabling instant updates without full page reloads. This minimizes latency and enhances the user experience, especially in applications requiring real-time data updates, such as chat applications or live dashboards.
Real-time Updates
Using SignalR, Blazor Server provides real-time communication between the server and the client, enabling instant updates without full page reloads. This minimizes latency and enhances the user experience, especially in applications requiring real-time data updates, such as chat applications or live dashboards.
Example:
@code {
private List<Item> items;
protected override async Task OnInitializedAsync()
{
items = await ItemService.GetItemsAsync(); // Fetch items from the server
}
}
In this example, when initializing a Blazor Server component, data fetching can be optimized to minimize network requests, thereby improving performance.
Blazor Web Assembly:
Near-Native Performance
Blazor WebAssembly applications achieve near-native performance by executing code directly within the browser using WebAssembly. This allows for faster load times and smoother interactions, as the code is compiled ahead of time and executed at close-to-native speed within the browser environment.
Offline Capabilities
Blazor WebAssembly applications can function offline once initially loaded. This is possible due to caching capabilities, allowing the application to run independently of a network connection after the initial download, further enhancing performance and user experience.
@code {
private List<Item> items;
protected override async Task OnInitializedAsync()
{
var response = await httpClient.GetAsync("https://api.example.com/items");
if (response.IsSuccessStatusCode)
{
items = await response.Content.ReadFromJsonAsync<List<Item>>();
}
}
}
Here, in a Blazor WebAssembly component, fetching data can be optimized by utilizing asynchronous HTTP requests efficiently, reducing load times and enhancing overall performance.
Security and Type-Safety:
This benefit in Blazor refers to its ability to enforce strong typing and enhance security measures, ensuring a safer and more robust web application development environment. Let’s explore this benefit in both Blazor Server and Blazor Web Assembly:
Blazor Server:
Server-Side Execution
Blazor Server performs most of its logic on the server-side, reducing the exposure of critical code and business logic to the client. This architecture helps in protecting sensitive information and logic, enhancing the security of the application.
Type-Safety
Being based on C# and .NET, Blazor Server benefits from the strong typing inherent in these languages. This type of safety reduces the likelihood of runtime errors, enhancing the overall security and stability of the application.
@code {
private int count;
private void IncrementCount()
{
count++; // Type-safe operation, ensuring count remains an integer
}
}
In this example, the increment operation (
count++
) benefits from C#’s type-safety, ensuring that count
remains an integer, reducing the risk of unintended type conversions or errors.Blazor Web Assembly:
Client-Side Sandboxing
Blazor WebAssembly applications run in a sandboxed environment within the browser, ensuring that they don’t have direct access to system resources or sensitive information on the client’s device. This sandboxing enhances the security of client-side execution.
Type-Safety
Similar to Blazor Server, Blazor WebAssembly also benefits from C#’s type-safety, reducing the chances of type-related vulnerabilities and ensuring a more secure application.
@code {
private int count;
private void IncrementCount()
{
count++; // Type-safe operation, preserving the integrity of count as an integer
}
}
Here, in a Blazor WebAssembly component, C#’s type-safety ensures that the
count
variable remains an integer, minimizing the risk of type-related security vulnerabilities.Conclusion:
In conclusion, Blazor stands out as a game-changer in web development, offering a compelling alternative to traditional JavaScript frameworks. By harnessing the power of C# and .NET, Blazor streamlines development, enhances performance, and facilitates the creation of robust, maintainable web applications. Whether it’s the seamless integration with existing .NET infrastructure or the efficiency of a component-based architecture, Blazor empowers developers to craft modern web experiences with ease. Embracing Blazor opens doors to a future where web development becomes more unified, performant, and enjoyable for developers.
By following these steps and harnessing the capabilities of Blazor, developers can create feature-rich, high-performance web applications while leveraging their existing C# expertise, ultimately delivering better user experiences on the web.
Do You Need more information?
For any further information / query regarding Technology, please email us at info@varianceinfotech.in
OR call us on +1 630 534 0223 / +91-7016851729, Alternately you can request for information by filling up Contact Us
Leave a Reply