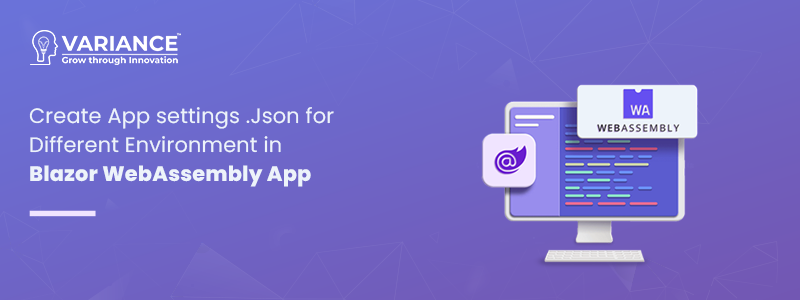
Create appsettings.Json for Different Environment
Our project structure looks like this:
In Blazor WebAssembly, you can achieve a similar behavior by merging the environment-specific appsettings.json file with the main appsettings.json file. Here’s how you can do it:
1. Create appsettings.json File: In your Blazor WebAssembly project, you can manually create an appsettings.json file in the wwwroot folder or another suitable location. You can use a text editor, an IDE, or the command line to create the file.
Example BlazorApp1/wwwroot/appsettings.json content:
2. This configuration would typically be used in a configuration file or settings file of your application. It’s common to have a configuration file that holds various settings for different environments. Developers can change the values in this file depending on the environment they are working with.
For example, if you’re working with a .NET Core or Blazor application, you might place this configuration in an appsettings.json file in your project’s root directory.
{
"Development-baseUrl": "https://localhost:8000",
"Stage-baseUrl": "http://apitest.com",
"Production-baseUrl": "http://apilive.com"
}
3. Next, make a new folder in wwwroot folder and name it “environment”. Inside this folder, create a Json file called “BlazorApp1/wwwroot/environment/env.config.json”.
In env.config.json file set Environment like below:
{
"Environment": "Stage"
}
Note: You can set the environment as per your requirements like Development, Stage, and Production
4. Now, create a “BlazorApp1/Model/EnvironmentModel.cs”.
namespace BlazorApp1.Model
{
public class EnvironmentModel
{
public string Environment { get; set; }
}
}
5. Now, create a BlazorApp1/Extension/WebAssemblyHostBuilderExtensions static class with GetEnvironment method.
using BlazorApp1.Model;
using Microsoft.AspNetCore.Components.WebAssembly.Hosting;
using System.Net.Http.Json;
namespace BlazorApp1.Extension
{
public static class WebAssemblyHostBuilderExtensions
{
public async static Task<EnvironmentModel> GetEnvironment(this WebAssemblyHostBuilder builder)
{
var httpClient = new HttpClient
{
BaseAddress = new Uri(builder.HostEnvironment.BaseAddress)
};
var environment = await httpClient.GetFromJsonAsync<EnvironmentModel>("environment/env.config.json");
return environment;
}
}
}
In above code GetEnvironment extension method helps retrieve environment-related configuration data from a JSON file in a Blazor WebAssembly application using an `HttpClient` to make an HTTP GET request. This can be useful for dynamically loading environment-specific settings during the application’s startup.
6. Configuration Program.cs file:
In a Blazor WebAssembly application, you can organize this code in the BlazorApp1/Program.cs file.
// Create a WebAssembly host builder
var builder = WebAssemblyHostBuilder.CreateDefault(args);
// Get and set up the environment and Base URL
var environment = await builder.GetEnvironment();
Console.WriteLine("environment {0}", environment.Environment);
var DefaultApi = builder.Configuration.GetValue<string>($"{environment.Environment}-baseUrl");
builder.Services.AddScoped(sp => new HttpClient { BaseAddress = new Uri(DefaultApi) });
Console.WriteLine("DefaultApi {0}", DefaultApi);
// Start building and running the application
await builder.Build().RunAsync();
- The program starts by trying to get information about the environment.
- It then prints the name of the environment to the console.
- The code retrieves a Base URL from the application’s configuration. The specific Base URL is determined by the environment and uses it to build the DefaultApi variable.
- It sets up a way to communicate with external services using an HttpClient. The HttpClient is configured with the previously obtained Base URL.
- Finally, the program prints the Base URL to the console.
Now, We can see that inside the appsettings.json file that I set stage URL is printed in the console.
Now, Alter the environment setting from “Stage” to “Production” and execute the application.
In the above picture, we can see the environment “Production” and DefaultApi is “http://apilive.com”.
Conclusion:
In conclusion, utilizing the appsettings.json file in a Blazor WebAssembly app offers a robust and flexible approach to managing configuration settings, particularly when catering to different environments. By structuring your app’s configuration using this file, you can easily adjust settings based on various deployment scenarios, such as development, testing, and production.
Setting different environments within the appsettings.json file empowers you to encapsulate specific configuration values for each environment, ensuring seamless transitions and consistent behavior across various deployment stages. Whether it’s database connection strings, API endpoints, feature toggles, or any other configuration parameter, this approach provides a centralized and manageable solution to maintain your app’s settings.
Do You Need more information?
For any further information / query regarding Technology, please email us at info@varianceinfotech.com
OR call us at +1 630 861 8283, You can request for information by filling up Contact Us







